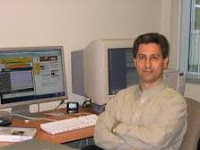
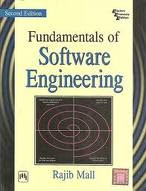
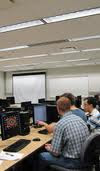
Fundamentals of Software Engineering
By Carlo Ghezzi, Mehdi Jazayeri, and Dino Mandrioli
Teaching points for each chapter
Here we list the important concepts of each chapter, without the supporting details. These are the points that each chapter attempts to teach. The students can best appreciate these points only after they have finished the course. You may want to use these points to reinforce the students’ understanding after they have finished each chapter. You may want to use these points in your teaching each chapter.
Chapter 1
· · Software engineering is the application of engineering principles to the building of software
· · Software engineering is concerned with the multi-person construction of multi-version software
· · The field of software engineering was born in 1968 in response to chronic failures of large software projects to meet schedule and budget constraints
· · Software engineering is often part of systems engineering, which is concerned with building a system composed of many components, one of which is software
· · Software engineering involves much more than programming
· · The main activities in software engineering are: requirements analysis, software design, coding, testing, deployment, and maintenance
Chapter 2
· · Software engineering is an intellectual activity and thus human-intensive
· · Software is built to meet a certain functional goal and satisfy certain qualities
· · Software qualities are either external or internal
· · External qualities include: correctness, performance, inter-operability, usability, reusability
· · Internal qualities include: maintainability, verifiability
· · Software processes also must meet certain qualities
· · Software process qualities include: timeliness, productivity, visibility
· · Software qualities are sometimes referred to as “ilities”
Chapter 3
· · Principles form the basis of methods, techniques, methodologies and tools
· · Principles stay constant as tools and techniques change and mature
· · Methodologies package specific tools and techniques to encourage a particular approach to software development
· · There are seven important principles that may be used in all phases of software development and in designing software processes
· · The seven principles we advocate are: rigor and formality, separation of concerns, modularity, abstraction, anticipation of change, generality, and incrementality
· · These principles are strongly related and mutually reinforcing
· · Modularity is the cornerstone principle supporting software design
Chapter 4
· · The architecture of a software system describes the structure of the system in terms of its components and their relationships. Modularization techniques are used to decompose a system into modules or components. The module decomposition may be used as the basis of work assignment to programmers and concurrent development.
· · A module’s interface describes the externally visible services provided by the module; the interface is a contract between the clients of the module and the implementers of the module. The implementers of the module must ensure that the module provides the services promised in its interface.
· · There are many relationships among modules of a system that are useful for understanding the system’s structure and behavior. Two important relationships are the USES relationship and the IS_COMPONENT_OF relationship.
· · Design is the activity of inventing a structure and architecture for a system. The result of a design activity is also called a design.
· · Any significant product, such as a document, must be designed. Examples of documents that we must design are requirements documents, specification documents, user manuals, and test plans.
· · An architectural design consists of a high level design and a low level design of the system. Design notations, both textual and graphical, are used to document the results of the architectural design.
· · Some prominent software design techniques are function-oriented, data oriented, and object oriented. Information hiding is a design technique based on the idea that each module encapsulates a single design decision. Information hiding is the basis of object oriented design.
· · The UML is a collection of design notations for describing object-oriented designs. It includes use case diagrams for describing functional requirements, class diagrams for describing static relationships among components, and sequence diagrams for describing dynamic interactions among components.
· · Concurrent software must deal with the presence of parallel threads of activity. Synchronization techniques are needed to mediate competing attempts to access and modify shared sources.
· · Distributed software must deal with not only concurrent activities but also physically distributed location of resources. Techniques are needed for locating and accessing remote resources and communicating with remote processes.
· · Design patterns capture the structure of common design and approaches to solving them. Each design pattern specifies the conditions under which the solution is effective. The model-view-controller is a design pattern for certain GUI problems.
· · An architectural style describes the kinds of components that may be used in an architecture and the allowed interaction paradigms among the components. Example architectural styles are: pipeline, blackboard, and event-based.
· · Component based software engineering promotes the construction of software based on assembling already existing components.
Chapter 5
· · Specification is a very broad term that means “definition”
· · We may write specifications in different phases of the software process; we may write different specifications for different purposes (a contract between user and producer, an interface between interacting modules); we may write different specification documents with different styles and languages, depending on their goals and readers
· · Just as other artifacts, specifications have their own qualities: among other qualities, they must be clear, precise, consistent, complete, and evolvable.
· · Specifications serve the purpose of verifying implementation correctness but must themselves be verified against the desired qualities. Such a verification of specifications is often called validation.
· · Specification styles can be classified according to two major categories:
o o Operational specifications (based on some abstract machine)
o o Descriptive specifications (focusing on system properties rather than on system modeling)
· · The formalizing of specifications enables automatic generation of test data, automatic generation of models for simulation, and automatic derivation and proof of properties and correctness.
· · Some important specification (semi)formalisms are:
o o Operational notations:
§ § Data Flow Diagrams
§ § Some UML diagrams
§ § Finite State Machines
§ § Petri Nets
o o Descriptive notations:
§ § Entity Relationship Diagrams
§ § Mathematical logic-based notations
§ § Algebraic notations
· · To be effectively used in practice, specification notations must include many features, primarily the ability to modularize large specifications.
· · Two important formal specification languages are:
o o Statecharts, based on Finite State Machines
o o Z, based on mathematical logic and set theory
Chapter 6
· · Verification of any activity whose results are important is necessary because humans make mistakes
· · Software verification cannot be restricted to checking that programs produce the expected results
· · All activities and artifacts produced during software development—and all their qualities—should be verified, including specifications and verification itself
· · Verification can exhibit quite different features. It may be:
o o Objective or subjective
o o Quantitative or qualitative
o o “Black or white” or “gray scale”
· · Two major approaches to verification are:
o o Experimentation or testing
o o Analysis
· · Testing does not provide absolute certainty about software correctness; nevertheless it is a major verification technique
· · Testing requires solid theoretical foundations to come up with reliable and general testing criteria
· · Testing can be classified into:
o o White-box or structural testing
o o Black-box or functional testing
· · Testing criteria may be described in terms of some kind of complete-coverage principle such as edge coverage, condition coverage, requirements specification coverage, and so on.
· · Functional testing can be strongly enhanced by formal specifications, for example, to derive test cases (semi)automatically.
· · Principles of testing may be applied also to object-oriented software.
· · Concurrent and real-time systems pose special needs and challenges for testing.
· · Analysis as a verification technique is complementary to testing. Analysis can be either informal or formal
o o Typical informal analysis techniques are code inspections and walkthroughs
o o Correctness proofs are a more sophisticated analysis technique based on formal specifications
· · Two techniques that aim to combine the features of testing and (formal) analysis are model checking and symbolic execution
· · Debugging is the activity of locating and fixing errors once they have been signaled by the verification activity
· · Verifying software qualities other than functional correctness and performance is a more difficult and often subjective task. Yet, for practical reasons, metrics are needed to measure such qualities. But…
o o Traditional statistical models that have proved effective for measuring reliability in traditional engineering fields are difficult to apply to software because of software’s special characteristics.
o o Even more controversial is measuring subjective qualities such as understandability, evolvability, etc.
Chapter 7
· · The life cycle of a software product begins with the inception of an idea for a product and goes through many stages, including requirements gathering and analysis, architecture design and specification, coding and testing, delivery and deployment, maintenance and evolution, and finally retirement.
· · A software process model is an attempt to organize the software life cycle by defining the activities involved in software production, the order of those activities, and the relationships among them.
· · The goal of a software process is to achieve predictability, productivity, high product quality, which in turn lead to the ability to plan time and budget requirements reliably.
· · Standardized and defined processes in production and manufacturing support automation of the processes and thus lead to reliability and efficiency. Software production is largely an intellectual activity and thus resists automation.
· · The original software process described in the 1960s is called the waterfall process and assumes a linear and sequential ordering of the activities, each activity resulting in a document that is delivered as input to the next activity; it is therefore characterized as document-driven.
· · Software processes are attempts at mastering the complexity of software development and thus rely on the principles of separation of concern, divide and conquer, and rigor and formality. The rigorous definition of the process leads to automatic tools and environments to support the process. The essence of a software process may be summarized by two questions: what shall we do next? And how long should we continue to do it?
· · Software processes may be used to define in great detail the steps to be followed in software development. Such detailed definitions are packaged as methodologies. Two well-known such methodologies are structured-analysis/structured-design (SA/SD) and Jackson system development method (JSD).
· · Alternatives to the waterfall process model have been proposed and used. Among the industrially relevant ones are:
o o Synchronize-and-stabilize popularized by Microsoft.
o o Unified process based on architectural design, object-oriented development with UML, and incremental delivery.
· · Configuration management is a discipline that deals with the disciplined management and control of change. It is a critically important part of any process, including software processes, in which many documents are produced and modified by large numbers of people.
· · Since a lot of software engineering is actually involved in software maintenance rather than new software development, special processes may be needed with special focus on the activities in the maintenance of legacy software.
Chapter 8
· · Software engineering projects involve many software engineers. Management is needed to coordinate the activities and resources involved in projects.
· · A project manager’s tasks are planning, resource acquisition, organization, project execution, project monitoring, and dealing with deviations from the plan.
· · The project manager’s challenge is to balance conflicting goals, and deliver a high-quality product with limited resources. The project manager’s task is to “Plan the work and work the plan.”
· · The primary challenge of software engineering management is to organize an activity that is fundamentally intellectual. This intellectual nature of software development complicates the traditional techniques for productivity measurement and therefore project planning in terms of cost and schedule estimation.
· · Two common methods for measuring software productivity are the number of source code lines and function points. Source code lines measure what is actually produced by software engineers and thus how much time they spent on the task; function points measure how much functionality is developed and thus how much of a user’s requirements have been addressed. Source code lines are easy to measure. Function points quantify something that is inherently difficult to measure.
· · Three tools that managers use to plan and monitor projects are: Work Breakdown Structures to understand the amount of work in terms of its constituent parts, GANT charts for resource planning, and PERT charts for activity and dependency planning.
· · An organization structure is used to structure the communication patterns among members of a team. The traditional team organization is hierarchical with a manager supervising a group or groups of groups. Other organizations have been tried in software engineering with some success. Some alternative team organizations are chief-programmer teams and democratic organizations. Chief-programmer teams are best when the solution may be understood and controlled by one chief architect; the democratic organization works best when the problem or solution is not well-understood and requires the contribution of several people to clarify it.
· · Quality standards have been developed to quantify the capability of software organizations. One such standard is the capability maturity model that categorizes the capabilities according to the five increasingly capable classes: initial, repeatable, defined, measured, and optimizing.
Chapter 9
· · Computer-aided software engineering tools and environments support software engineering teams by automating routine tasks, keeping track of dependencies, visualizing information, maintaining databases, supporting and enforcing methodologies and processes, and supporting and controlling group interactions.
· · Tools may support single activities or a related set of activities, individual engineers or team(s) of engineers, single methods or groups of related methods, single process phases or entire process.
· · Some important classes of tools, used in different phases of the life cycle, are: editors, linkers, generators, interpreters, debuggers, analyzers, tracking tools, reverse engineering tools, and management tools.
· · Two essential classes of tools are build tools and configuration management tools. Build tools such as make and its derivatives automate the generation a product from a declarative description of its structure and dependencies. Configuration management tools such as cvs support disciplined sharing and parallel development of documents by enforcing protocols for applying changes to documents.
· · Tools may be compared along many dimensions, including interactivity, interface format, single- vs. multi-user, static vs. dynamic, language dependency, network awareness, and level of formality.
· · Tools will evolve to support new technologies (e.g. the Internet and mobile devices), new processes (e.g. open software), and new methods (e.g. model checking).
Chapter 10
· · The software engineering field has made enormous progress since its “crisis” days of the late 1960s. We now find software in every facet of society: transportation, government, factories, consumer products,
· · Requirements for software applications and systems are growing in complexity and will continue to do so due to the emergence of new application areas with critical requirements
· · Reliability of software is an increasingly important quality and places special responsibility on the shoulders of software engineers
· · The information revolution spurred by the Internet has also affected software engineering. The software is both an enabling technology for the Internet and may rely on the Internet to provide new possibilities for software engineering.
· · The code of ethics developed by the ACM and IEEE is a framework that helps software engineers confront and address ethical issues faced in practice.
· · Using the principle of separation of concerns, the code of ethics is divided into eight separate concerns dealing with such concerns as the public interest, the product quality, and relationships with colleagues, clients, and employers.
Case studies
A. A. Automating a law office
a. a. Good intuition and technical skills are not enough to guarantee success
b. b. Marketing analysis and process organization must be planned carefully before committing to the project
c. c. Changes in schedules and project sourcing after serious problems are encountered can be difficult and expensive, if not impossible to implement
B. B. Building a family of compilers
a. a. Good engineers are essential to the success of a project
b. b. Use of standard test suites helps obtain objective measures of the completion status of a project
c. c. Transparency of process helps entire organization in planning
d. d. Revising a schedule on the basis of objective data helps the schedule become more realistic
e. e. Reuse of large software components on the basis of clear specifications is possible and advantageous
C. C. Incremental delivery
a. a. Prototyping is possible
b. b. A throw-away prototype may be used by clients to start on their project while they await the final product
c. c. A throw-away prototype enables parallel development by clients and developers
d. d. A throw-away prototype may be used to prune an initial set of requirements on the basis of actual usage and need for features
D. D. Applying formal methods in industry
a. a. Formal methods are effective even in practical cases and their use confirms the expectations in terms of increased reliability and reduced development and verification costs which compensate the increased effort in the specification phase
b. b. Nevertheless industrial environments are still reluctant to systematically adopt them, even after successful pilot projects
c. c. A major reason for this situation may be due to the special training needs they exhibit and the consequent organizational efforts and economic investments
d. d. To overcome such difficulty a “lightweight approach” may be possible which exploits the incrementality principle
0 comments:
Post a Comment